In this article we are going to learn about the websocket API and its protocols. Here is what we are going to learn
- Websocket overview
- Websocket comparison VS HTTP and Key advantages of Websockets
- Detailed understanding of how websockets actually work
- Websocket handshake, data frames and control frames
- Simple Code Examples
- How to set up a websocket server
- How to set up a websocket client
- Advance topics in websockets
- Subprotocols, extensions and binary data
- Real time chat with Websockets
Websocket overview
Websocket protocol is a technology that allows for bi directional communication and persistant connection between servers and clients
It offers a single, long lived connection that is a more efficient alternative to HTTP for real time web apps
Here are some fo the freatures of websockets connections
1. Full duplex connection
Websockets have a full duplex connection which means that the data can flow in both the directions simultaneously.
2. Single long lived connection
One the connection is established, the connection remains live until this is disconnected. unlike HTML where each request opens a new connection is received.
3. Websocket handshake
Websocket connections begin with a handshake that is initiated by the client. This request form the client is called an upgrade request as it asks the server to upgrade the HTTP request to the websocket request. If the server supports websocket protocol then the server upgrades the conncetion to a websocket connection
4. Url Scheme
Websockets connections have a specific websocket url. There are two types of websocket schemes these are ws://
for unencrypted connections and wss://
for encrypted (TLS/SSL) encrypted connections
5. Data frames
Commuication in websockets is done through messages, which are transmitted in data frames. Frames contain binary data allowing for transmission for various kinds of data through websocket protocol
6. Compatibility
Websockets are compatible with all the major web browsers, providing a consistent experience for developers
7. use cases
Websockets are good for use-cases where is a need for bi drirectional communications such as real time chat, online games, live sports updates, financial trading platforms and any other systems that require real time communication
Websockets vs HTTP
Websockets and HTTP both communication protocols are fundamentally different from each other in various aspects
Each protocal has its own sets of advantages and disadvatages for perticular use cases.
Websockets are generally preffered and more suitanble for real time communication. Here are some of the differences between websockets and HTTP
1. Connection persistence
Websockets: In websockets the connection is persistent, untill and unless the connection is disconnected by either parties.
HTTP: In the request-response model of HTTP, a new connection is open for each request. There are workarounds for this as well like keep-alive header or the HTTP/2 feature of multiplexing over a single connection. But HTTP basically and fundamentally is a req response model.
2. Data transfer efficiency:
Websockets: are more efficient in terms of data transfer because they lack headers. Headers provide more baggage in terms of added message load which increases latency and requires more bandwidth.
HTTP: Each HTTP request includes headers which can add message load and hence it is resource intensive for small and frequent data transfer requests.
3. Suitability for Use Cases
Websockets: are good for real time communication apps like chat, online gaming, finance and stock market updates
HTTP: HTTP is good for document centric apps where a client initiates the connection and the server replies and that's the end of a transaction. This includes loading web pages, REST APIs and form submission
4. Compatibility and support
Websockets: Implementing websockets involves handling a persistant connection explicitly. Websockets are universally supported across all modern browsers but handling it across firewall rules and having a fallback mechanism could potentially add complexity
HTTP: HTTP being a older protocol is universally acceptable in older browsers and systems as well. HTTP has a vast libraries and tools to optimize its usage and works across firewalls and is reponsable for most interactions across the web
5. Security
Websockets: With regards to security websockets use the ws://
and wss://
for unencrypted and encrypted connections. Thus you can easily encrypted the connections while maintaining a secure and persistant connection and avoid hacks like cross site websocket hijacking and other such malicious tricks to steal data.
HTTP: HTTP uses https://
to encrypted client server communications using TLS/SSL. HTTPS has well established security and communication protocols including CORS, content security policies and secure cookies
6. Protocol overhead and Latency
Websockets: Once the initial handshake is performed there is minial overhead in message delivery. This isimportant for performance and real time connectivity
HTTPS: Does have more overhead than websockets but is the standard protocol that can be used in a variety of use-cases
Detailed understanding of how the websocket protocol actually works
Websocket handshake:
The websocket handshake is the process through which websocket connection is established.
To establish a websocket connection the client sends a HTTP request with some special headers. These headers are
Upgrade
header: Asks the server to upgrade to connection to websocket from httpConnection
header: is set tpUpgrade
so as to signal to the server that client wants to establish a websocket connectionSec-WebSocket-Key
: A base64-encoded random value key that the server will use to help form a response to let the client know that it supports the websocket protocolSec-WebSocket-Version
: This specifies the websocket version that the client is using
If the server supports the websockets and accepts the connection it sends a http response with the HTTP status code 101 Switching Protocols
and includes its own special headers like
Upgrade
: Server sends this header to indicate that the connection is upgraded to websocket connectionConnection
This is set to UpgradeSec-Websocket-Accept
A hash is derived from the key that the client sent and a specific GUID that is defined in the websocket protocol
Once the handshake is established the connection is switched to websocket connection
Data Frames
- Control frames
- Security aspects
Simple Code Examples
In this section we are going consider the workings of the websocket with the means of a simple example
We are going to create a simple websocket server and a client and establish communication between then and send messaging.
We are going to be building a proof of concept here to test out how the websocket protocol works
How to set up a websocket server
first create a new directory called websocket server. then initalize a new project and name it websocket-server
and set the entry point to index.js
like
npm init websocket-server
next we are going to use the websocket library ws
for websocket connections. Install the websocket library ws like
npm install ws
Then write the below code to create a websocket server
const WebSocket = require('ws');
const wss = new WebSocket.Server({ port: 4000 });
wss.on('connection', function connection(ws) {
console.log('A new client connected.');
ws.on('message', function incoming(message) {
console.log('received: %s', message);
// Send the message back to the client
ws.send(`Server received: ${message}`);
});
ws.on('close', () => {
console.log('Client is disconnected.');
});
});
console.log('WebSocket server is running on ws://localhost:4000');
Here we have created a websocket server. start the server with
node index.js
The websocket server listens on port 4000 then when a client connects to the server, it logs a message, listens to the incoming message and then sends this message back to the client.
How to set up a websocket client
We are going to build the websocket client. We are going to create a simple websocket client with HTML and js to connect to the websocket server
In the same directory where we created the server.
there create a new file and name it index.html and in the index.html write the following html and js code to connect it to the server that we created
<!DOCTYPE html>
<html>
<head>
<title>WebSocket Client</title>
</head>
<body>
<script>
const ws = new WebSocket('ws://localhost:4000');
ws.onopen = function() {
console.log('Connected to the websocket server');
ws.send('send a message!');
};
ws.onmessage = function(event) {
console.log(`Message from server: ${event.data}`);
};
ws.onclose = function() {
console.log('Disconnected from the websocket server');
};
ws.onerror = function(error) {
console.log(`WebSocket error: ${error}`);
};
</script>
</body>
</html>
to test out the client server connection. Open the client file in a web browser and then the following steps will take place
- the client will connect to the server
- the server is listning to the messages from the client
- the client will send a message to the server
- the server will recieve the connection and the message and log the message to the console and also send the message back to the client
- which in turn will log the message to its console
you can see this in the screen shots below
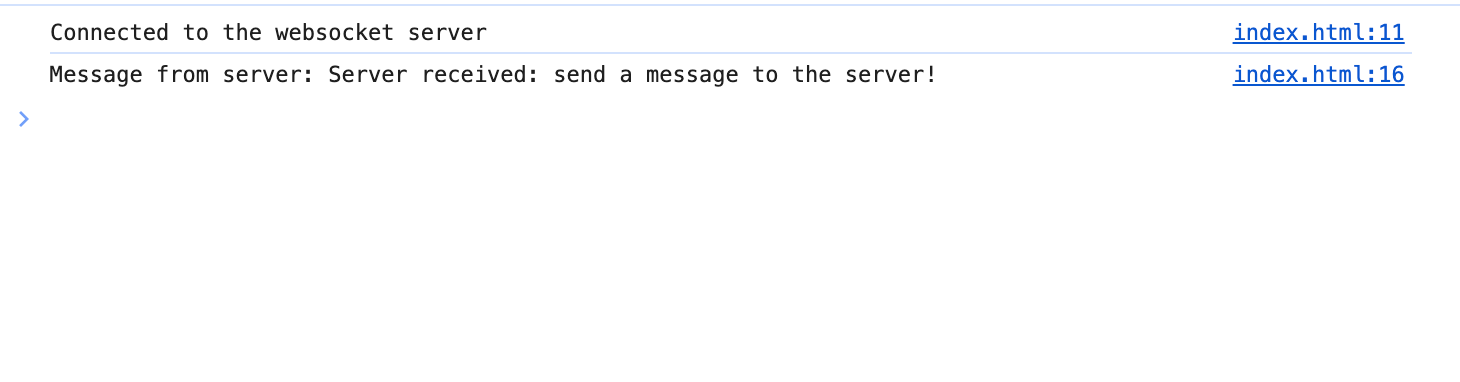
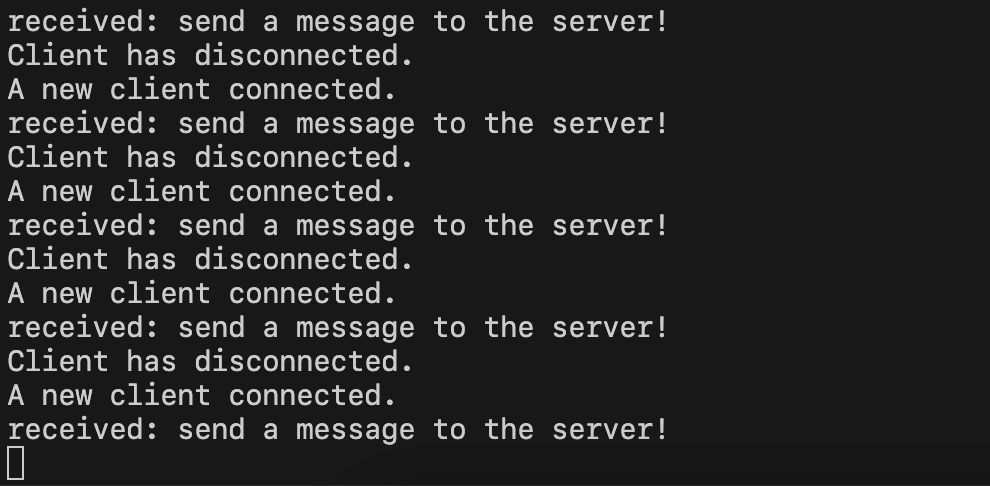
Advanced topics in websocket: Subprotocols, extentions and binary data
In this section we are going to learn about the advanced topics of websocket and explore its functionality in building real time web applications
1. Subprotocols
Websockets sub protocols are a way to agree on a specific protocol for a specific type of data between client and server
for example if you are building an application that requires different messaging protocols to send different types of data like JSON for text messages and a custom binary protocol for image and video data
you can seprate these data formats and ensure that both client and server know what kind of data is being transmitted and how to process that specific kind of data
This is done using the Sec-Websocket-Protocol
header. the client can specify in this header the kinds the sub protocols the client wantst to use.
This is done when establishing a websocket connection with the server. The server can then speicify if any specific protocol it is going to use in the handshake response, thus indicating the chosen sub protocol for that specific websocket connection.
2. Extensions
Websocket extentions are a popular choice for extending the websockets functionality. They provide additional features lije meaage compression which can reduce the amount of data that is sent. There are other extentions as well which adds other types of funcitonalities to websockets
The extentions are negotiated in the handshake like sub protocols are, for the extentions there is the Sec-Websocket-extentions
header
Let us consider an example: The per message deflate extention is a popular choice for compressing the websocket data and sending it, thus reducing bandwidth requirements
3. Binary data
Websockets supports both text and binary data making it functional to send a wide variety of data formats. Any data including image and video that can be converted into binary data can be sent through websockets.
Real time chat with websockets
If you are looking for a real time chat solution then building one yourself with websockets is a difficult one. let alone being able to scale it to millions of users
One of the solutions could be to use the pre built chat api solution from DeadSimpleChat that is cost effective and scaleable to millions of concurrent online users
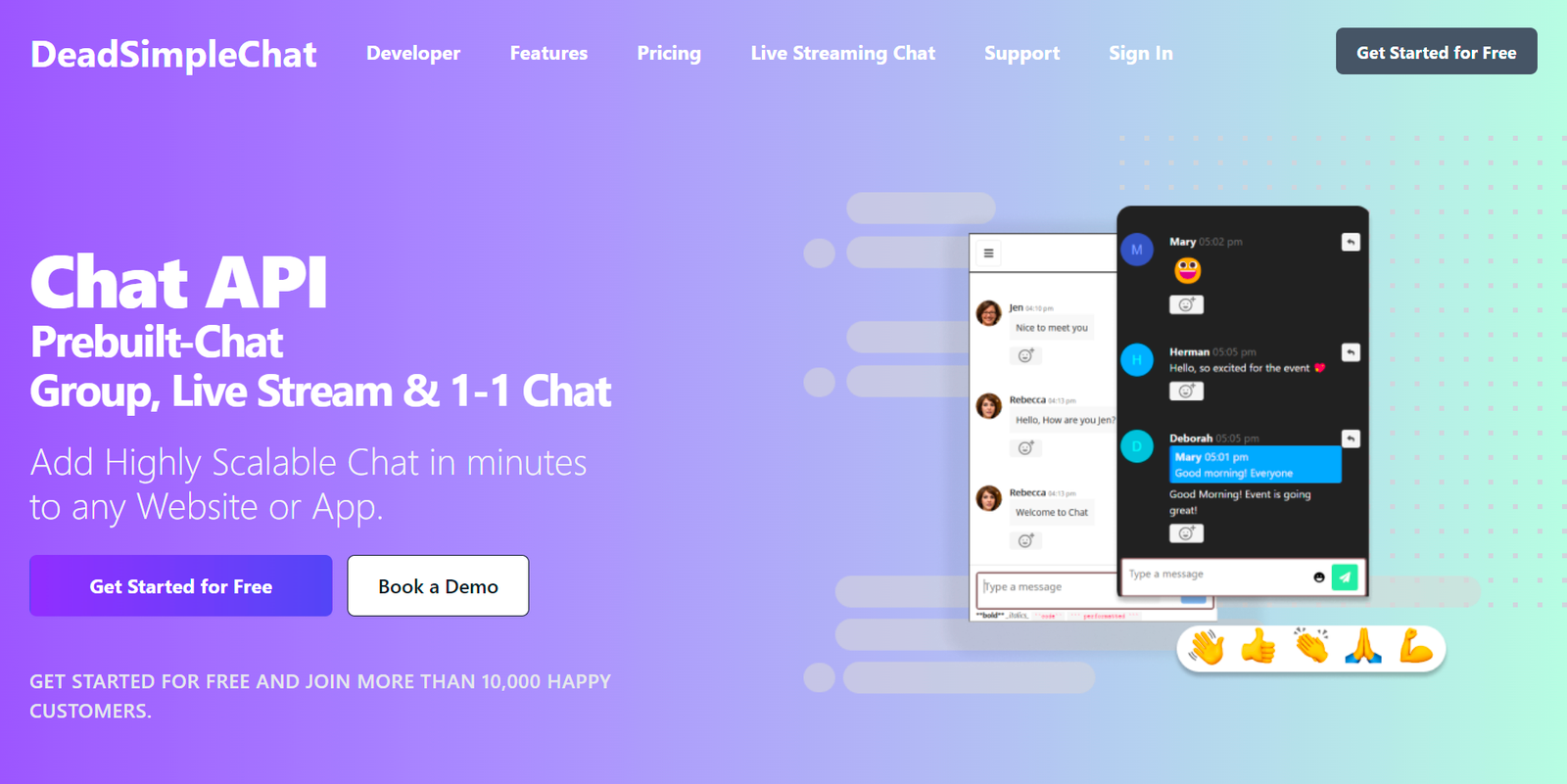
Chat API for your website or app
DeadSimpleChat is an Chat API provider
- Add Scalable Chat to your app in minutes
- 10 Million Online Concurrent users
- 99.999% Uptime
- Moderation features
- 1-1 Chat
- Group Chat
- Fully Customizable
- Chat API and SDK
- Pre-Built Chat
Also consider Metered TURN server
Metered TURN servers
- API: TURN server management with powerful API. You can do things like Add/ Remove credentials via the API, Retrieve Per User / Credentials and User metrics via the API, Enable/ Disable credentials via the API, Retrive Usage data by date via the API.
- Global Geo-Location targeting: Automatically directs traffic to the nearest servers, for lowest possible latency and highest quality performance. less than 50 ms latency anywhere around the world
- Servers in 12 Regions of the world: Toronto, Miami, San Francisco, Amsterdam, London, Frankfurt, Bangalore, Singapore,Sydney (Coming Soon: South Korea, Japan and Oman)
- Low Latency: less than 50 ms latency, anywhere across the world.
- Cost-Effective: pay-as-you-go pricing with bandwidth and volume discounts available.
- Easy Administration: Get usage logs, emails when accounts reach threshold limits, billing records and email and phone support.
- Standards Compliant: Conforms to RFCs 5389, 5769, 5780, 5766, 6062, 6156, 5245, 5768, 6336, 6544, 5928 over UDP, TCP, TLS, and DTLS.
- Multi‑Tenancy: Create multiple credentials and separate the usage by customer, or different apps. Get Usage logs, billing records and threshold alerts.
- Enterprise Reliability: 99.999% Uptime with SLA.
- Enterprise Scale: With no limit on concurrent traffic or total traffic. Metered TURN Servers provide Enterprise Scalability
- 50 GB/mo Free: Get 50 GB every month free TURN server usage with the Free Plan
- Runs on port 80 and 443
- Support TURNS + SSL to allow connections through deep packet inspection firewalls.
- Support STUN
- Supports both TCP and UDP
Thank you for reading the article. I hope you liked it