In this article we are going to learn how we can automatically restart the nodejs application with nodemon.
Here is what we are going to learn in this article
- What is Nodemon?
- Installation and basic setup
- Setting an example Express Js project with Nodemon
- Advanced Configuration
- Containerization and NodeMon
- Debugging with NodeMon
Nodemon is a utility that monitors for any changes in your code base and restarts your server
This utility is primarily used in the development of NodeJs applications. Let us consider how to install and setup the nodemon with your express and nodejs project
Installation and basic setup of Nodemon
There are two ways you can install the nodemon. You can either install the nodemon globally in your machine or install it only in your project.
- Global installation
you can install the nodemon globally using the following command. By installing it globally all the programs running on your machine will have access to nodemon
sudo npm install -g nodemon
2. Project Specific installation
If you do not want to pollute your machine with unnecessary software, you can also install nodemon in specific projects.
Doing this the nodemon is contained and available only for those programmes
npm install --save-dev nodemon
Setting an example Express Js project with Nodemon
In this section let us setup an expressjs project with nodemon to see how it works.
- initialize your project
Create a new directory and name it node-express
, cd
in it and then initialize the project with npm init -y
mkdir my-node-project
cd my-node-project
npm init -y
then install the dependencies including expressjs
and nodemon
npm install express
npm install nodemon
then create an index.js
file and write the below code
const express = require('express');
const app = express();
app.get('/', (req, res) => {
res.send('Hello World!');
});
app.listen(3000, () => {
console.log('Application is running on http://localhost:3000');
});
lastly start the app using nodemon like
nodemon index.js
you can see the app running on localhost://3000 like
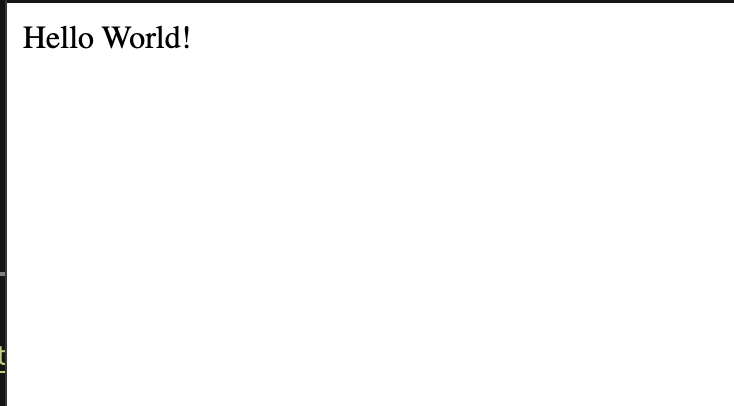
Advanced NodeMon Configuration
Configure nodemon to ignore certain files and folders
You can configure nodemon to ignore changes in specific files and folders. For example you want a folder that has assets, you do not want to restart server whenever there are changes to that folder.
To do this you need to create a nodemon.json
file in your root directory and list the files and folders.
{
"ignore":["README.md", "node_modules/*"]
}
using nodemon.json
for complex configurations
For more detailed configuration, such as specifying watched file extensions or if you want to set env variables nodemon.json
files is the way to go
Here is a sample complex configuration for your reference
{
"watch": ["src/"],
"ext": "js,ts",
"ignore": ["src/tests/"],
"env": {
"NODE_ENV": "development"
},
"delay": 1000
}
This configuration watches the src/
directory and restarts for changes in the js
and the ts
files
the configuration also ignores the changes in the src/tests/
directory, set the NODE_ENV
to development
and wait for 1000 miliseconds
Containerization and NodeMon
You can integrate nodemon with docker container as well. You can restart the nodejs app within docker container. here is how you can do this
- Dockerfile Configuration
- Create a
Dockerfile
for your Node.Js app - Install the nodemon globally in your docker container, this is probably the easier way. Or you can include it as a dependency in your development project as well in the docker container
- Set up the docker container to run the app using Nodemon. You can use this by defining the start command with nodemon in the
CMD
directive of yourDockerfile
Let us consider a docker example
FROM node:latest
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
CMD ["nodemon", "./src/app.js"]
Using Docker compose for ease of development
- You can use Docker Compose to manage your app services
- you can also mount your root project directory within the Docker container to enable the real time syncing of changes
Best Practices for using Docker in Development environment
- Make sure that local development environment is exactly looks like the Docker environment.
- Using volume mounts to sync source code between your host and container, allowing NodeMon to restart the app upon detecting changes
Debugging with Nodemon
Nodemon can be easily configured to work with nodejs built-in debugger or other debugging tools like chrome devtools or VSCode
You can do this by forwarding appropriate arguments to the NodeJs process
- Debugging config in
nodemon.json
You can specify the nodejs debugging arguments in the exec
command int he nodemon.js
file
Let us consider this example configuration
{
"exec": "node --inspect=9229 src/app.js"
}
for VS code you can setup a .vscode/launch.json
file to attach to the node process.
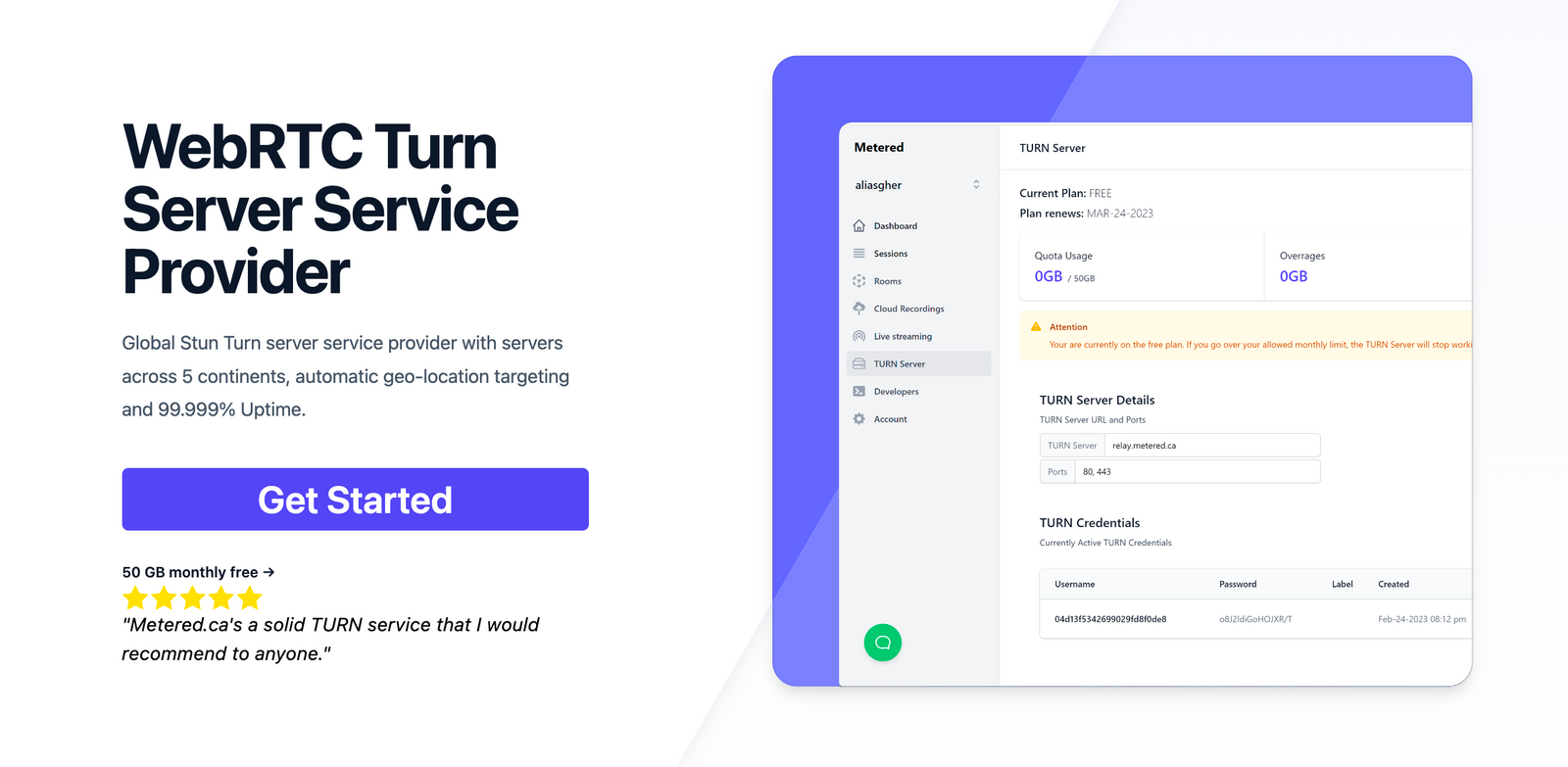
Metered TURN servers
- API: TURN server management with powerful API. You can do things like Add/ Remove credentials via the API, Retrieve Per User / Credentials and User metrics via the API, Enable/ Disable credentials via the API, Retrive Usage data by date via the API.
- Global Geo-Location targeting: Automatically directs traffic to the nearest servers, for lowest possible latency and highest quality performance. less than 50 ms latency anywhere around the world
- Servers in 12 Regions of the world: Toronto, Miami, San Francisco, Amsterdam, London, Frankfurt, Bangalore, Singapore,Sydney, Seoul
- Low Latency: less than 50 ms latency, anywhere across the world.
- Cost-Effective: pay-as-you-go pricing with bandwidth and volume discounts available.
- Easy Administration: Get usage logs, emails when accounts reach threshold limits, billing records and email and phone support.
- Standards Compliant: Conforms to RFCs 5389, 5769, 5780, 5766, 6062, 6156, 5245, 5768, 6336, 6544, 5928 over UDP, TCP, TLS, and DTLS.
- Multi‑Tenancy: Create multiple credentials and separate the usage by customer, or different apps. Get Usage logs, billing records and threshold alerts.
- Enterprise Reliability: 99.999% Uptime with SLA.
- Enterprise Scale: With no limit on concurrent traffic or total traffic. Metered TURN Servers provide Enterprise Scalability
- 5 GB/mo Free: Get 5 GB every month free TURN server usage with the Free Plan
- Runs on port 80 and 443
- Support TURNS + SSL to allow connections through deep packet inspection firewalls.
- Support STUN
- Supports both TCP and UDP
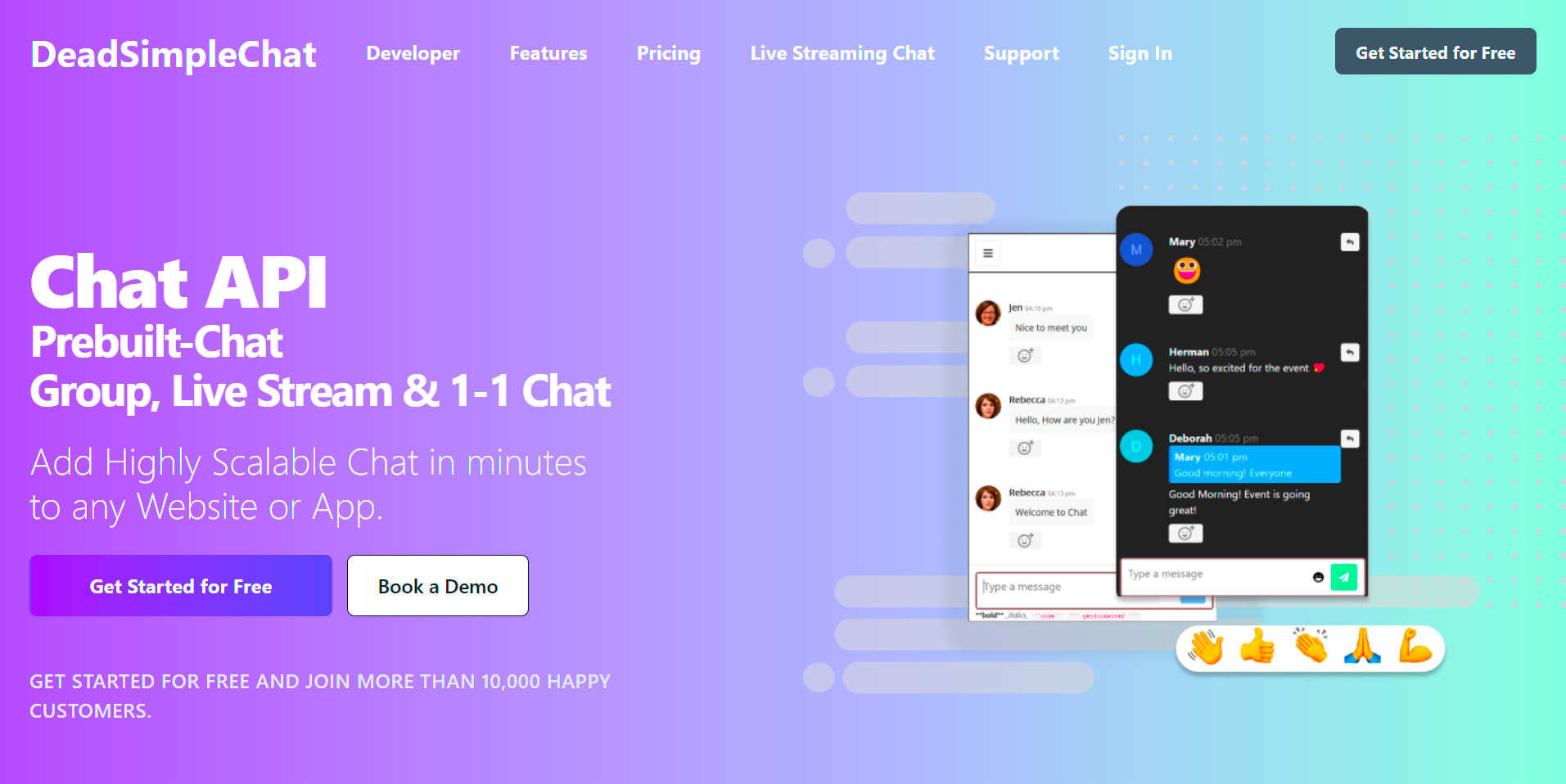
Need Chat API for your website or app
DeadSimpleChat is an Chat API provider
- Add Scalable Chat to your app in minutes
- 10 Million Online Concurrent users
- 99.999% Uptime
- Moderation features
- 1-1 Chat
- Group Chat
- Fully Customizable
- Chat API and SDK
- Pre-Built Chat
Here are some of our other article that might be interest to you:
- Best NodeJs Frameworks for Backend development in 2024
- Async/Await in TypeScript: A step by step guide
- How to Undo the Most Recent Local Commits in Git?
- Building a Group Video Chat App with WebRTC, PHP Laravel & JavaScript
Conclusion
In this article we have learned how we can use nodemon in development to automatically restart servers.
This is especially useful when you are developing an app and you need to see how the code changes are working without manually restarting the server again and again
Keep in mind though nodemon is for development use only, using nodemon in production poses security chanllenges and it is strongly advised not to use it.